Delving Deeper into Microsoft PowerApps: Operators and Functions (Part 2)
Understanding Operators in PowerApps
In Microsoft PowerApps, operators are essential for performing operations on data. They help manipulate data types, perform calculations, and facilitate logical operations. Throughout this section, we will categorize operators into various types: arithmetic, comparison, logical, and special operators.
Arithmetic Operators
Arithmetic operators allow you to perform mathematical operations. Below are some of the essential arithmetic operators:
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus (remainder of a division)
Example:
Result = 5 + 10 - 3 * 2 / 4; // The output will be 12.5
Comparison Operators
Comparison operators are used to compare two values and return a boolean result (true/false). Here’s a summary of the comparison operators in PowerApps:
=
: Equal to!=
: Not equal to>
: Greater than<
: Less than>=
: Greater than or equal to<=
: Less than or equal to
Example:
IsGreater = 10 > 5; // The output will be true
Logical Operators
Logical operators help in combining multiple conditions. The operators available are:
And
: Returns true if both conditions are true.Or
: Returns true if at least one of the conditions is true.Not
: Reverses the result of a condition (true becomes false and vice versa).
Example:
IsValid = (5 > 3) And (3 < 10); // The output will be true
Special Operators
PowerApps also includes specialized operators that have specific functions:
in
: Checks if a value exists within a collection or a table.- : Checks for the exact match of a value in a collection (case-sensitive).
Example:
IsInCollection = "item1" in ["item1", "item2", "item3"]; // The output will be true
Exploring Functions in PowerApps
PowerApps features a variety of functions to manipulate data effectively. Functions are categorized based on their functionality: Text, Number, Logical, Date and Time, Statistical, and Navigation functions.
Text Functions
Text functions are helpful for manipulating string values. Below are essential text functions:
LEN
: Returns the length of a given string.LOWER
: Converts a string to lowercase.UPPER
: Converts a string to uppercase.LEFT
: Extracts a specified number of characters from the left side of a string.RIGHT
: Extracts a specified number of characters from the right side of a string.MID
: Extracts a substring from a string, starting at a specified position.PROPER
: Capitalizes the first letter of each word in a string.SUBSTITUTE
: Replaces occurrences of a specified substring with another substring.REPLACE
: Replaces a substring with a different substring based on position.TRIM
: Removes unnecessary spaces from both ends of a string.TRIM ENDS
: Only removes spaces from the ends of a string.VALUE
: Converts a text string that represents a number into a numeric value.TEXT
: Converts a number or date/time value to a formatted string.CONCATENATE
: Joins together two or more strings into one.SPLIT
: Splits a string into a table based on a specified delimiter.
Example:
TextLength = LEN("PowerApps"); // The output will be 9
LowerCase = LOWER("HELLO WORLD"); // The output will be "hello world"
UpperCase = UPPER("hello world"); // The output will be "HELLO WORLD"
LeftText = LEFT("PowerApps", 5); // The output will be "Power"
Number Functions
Number functions are used to perform calculations and manipulations on numeric data. Important number functions include:
ABS
: Returns the absolute value of a number.MOD
: Returns the remainder after a number is divided by a divisor.POWER
: Raises a number to a specified power.SQRT
: Returns the square root of a number.ROUND
: Rounds a number to the nearest integer.ROUNDUP
: Rounds a number up, away from zero.ROUNDDOWN
: Rounds a number down, towards zero.INT
: Rounds a number down to the nearest integer.TRUNC
: Truncates a number to an integer (removes the fractional part).RAND
: Generates a random number between 0 and 1.RANDBETWEEN
: Generates a random integer within a specified range.VALUE
: Converts a text string representing a number into a numeric value.
Example:
AbsoluteValue = ABS(-5); // Output: 5
Remainder = MOD(10, 3); // Output: 1
Squared = POWER(2, 3); // Output: 8
SquareRoot = SQRT(25); // Output: 5
RoundedNumber = ROUND(3.14); // Output: 3
RoundedUp = ROUNDUP(3.14); // Output: 4
RoundedDown = ROUNDDOWN(3.14); // Output: 3
Date and Time Functions
Date and time functions handle date and time values. These functions are crucial for creating dynamic and time-sensitive applications.
TODAY()
: Returns the current date.NOW()
: Returns the current date and time.DATE(Year, Month, Day)
: Creates a date value from year, month, and day components.DAY(Date)
: Extracts the day from a date value.MONTH(Date)
: Extracts the month from a date value.YEAR(Date)
: Extracts the year from a date value.WEEKDAY(Date)
: Returns the day of the week as a number (1 for Sunday, 2 for Monday, etc.).WEEKDAYNAME(Date, abbreviated)
: Returns the name of the day of the week (abbreviated or full name).WEEKNUMBER(Date)
: Returns the week number of the year.DATEVALUE(DateString)
: Converts a text string representing a date into a date value.DATEADD(Date, Number, Units)
: Adds a specified number of units (days, months, years) to a date.DATEDIFF(StartDate, EndDate, Units)
: Calculates the difference between two dates in specified units.TIME(Hour, Minute, Second)
: Creates a time value from hour, minute, and second components.HOUR(Time)
: Extracts the hour from a time value.MINUTE(Time)
: Extracts the minute from a time value.SECOND(Time)
: Extracts the second from a time value.TIMEVALUE(TimeString)
: Converts a text string representing time into a time value.DATETIMEVALUE(DateTimeString)
: Converts a text string representing a date and time into a date and time value.
Example:
TodaysDate = TODAY();
CurrentDateTime = NOW();
NextWeek = DATEADD(TODAY(), 7, Days);
DaysBetween = DATEDIFF(TODAY(), DATE(2024,1,1), Days);
Statistical Functions
Statistical functions enable data analysis within your PowerApps. Key statistical functions include:
MAX(Column)
: Returns the maximum value in a specified column of a table.MIN(Column)
: Returns the minimum value in a specified column of a table.SUM(Column)
: Returns the sum of values in a specified column of a table.AVERAGE(Column)
: Returns the average of values in a specified column of a table.COUNT(Column)
: Counts the number of non-blank values in a specified column of a table.COUNTA(Column)
: Counts the number of all values (including blanks) in a specified column of a table.COUNTROWS(Table)
: Counts the number of rows in a table.COUNTIF(Table, Condition)
: Counts the number of rows in a table that meet a specified condition.
Example:
MaxValue = MAX(MyTable, ValueColumn);
MinValue = MIN(MyTable, ValueColumn);
TotalValue = SUM(MyTable, ValueColumn);
AverageValue = AVERAGE(MyTable, ValueColumn);
RowCount = COUNTROWS(MyTable);
Where `MyTable` is a table and `ValueColumn` is a column containing numeric values. Remember to replace these with your actual table and column names.
Navigation Functions
Navigation functions control the flow of your app, managing screen transitions and user interactions. These are essential for creating a user-friendly experience.
Navigate(ScreenName [, ScreenTransition])
: Navigates to a specified screen. `ScreenTransition` is an optional parameter specifying the transition animation (e.g., `ScreenTransition.None`, `ScreenTransition.Fade`, `ScreenTransition.Cover`).Back()
: Navigates back to the previous screen.Exit()
: Closes the app.
Example:
// Navigate to the "DetailsScreen" with a fade transition.
Navigate(DetailsScreen, ScreenTransition.Fade);
// Go back to the previous screen.
Back();
// Exit the application.
Exit();
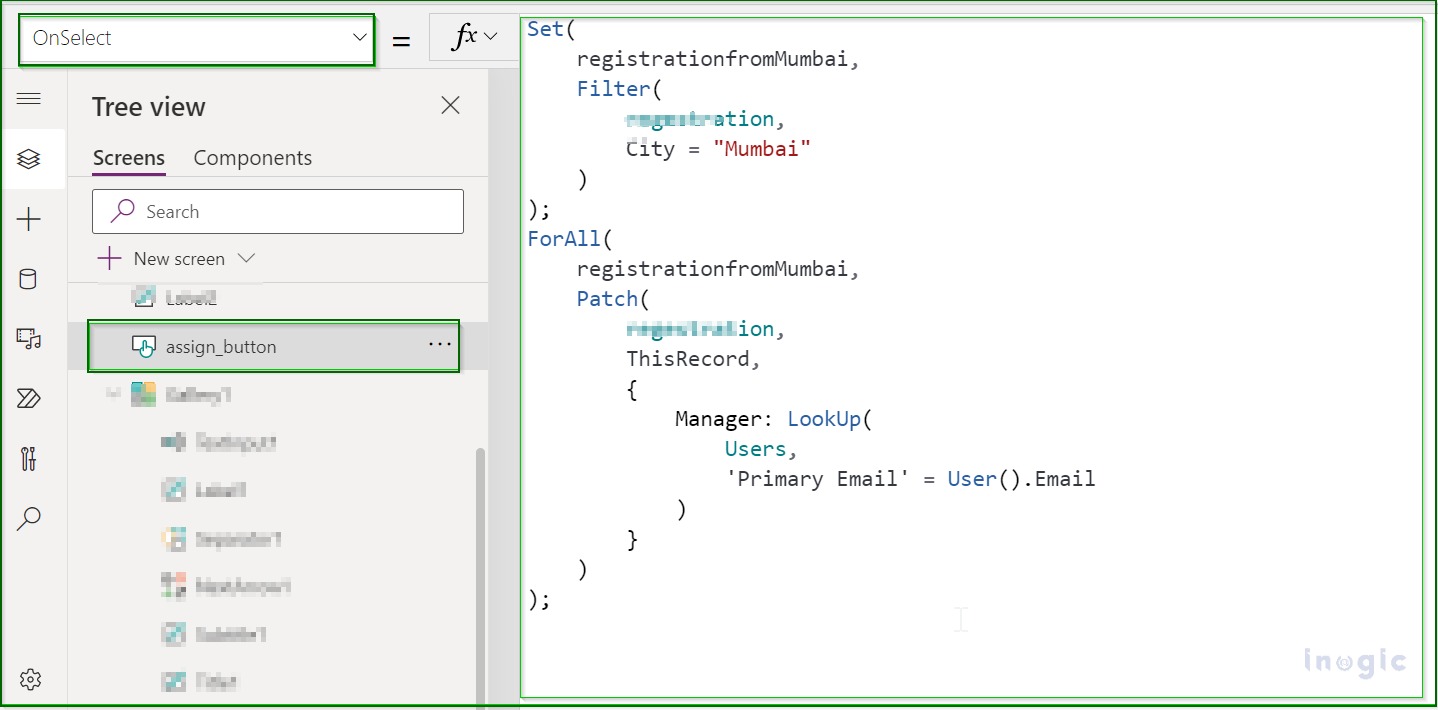
Advanced Usage and Best Practices
Mastering PowerApps involves understanding how to effectively combine operators and functions to achieve complex logic. Consider these best practices:
- Data Validation: Always validate user inputs to prevent errors and ensure data integrity. Use functions like `IsBlank`, `IsNumber`, `IsMatch` to check the validity of inputs before processing.
- Error Handling: Implement error handling to gracefully handle unexpected situations. Use `IfError` function to handle potential errors during calculations or data retrieval.
- Efficiency: Optimize your formulas for efficiency. Avoid unnecessary calculations or redundant function calls. Break down complex formulas into smaller, more manageable parts.
- Comments: Add comments to your formulas to improve readability and maintainability. This is especially important for complex formulas.
- Debugging: Utilize the debugging tools in PowerApps Studio to troubleshoot issues and identify errors in your formulas.
By understanding and applying these concepts, you can build sophisticated and robust applications using Microsoft PowerApps. Remember to consult the official Microsoft PowerApps documentation and TechNet articles for the most up-to-date information and detailed examples.
Microsoft Power Fx OverviewMicrosoft Power Fx Reference